Bơ rất tốt nhưng nên ăn bao nhiêu trái một ngày?-Soi cầu đá gà 8KBET
8KBET - Tin Tức và Trò Chơi Điện Tử cung cấp thông tin nổi bật và cập nhật hàng ngày về các trò chơi điện tử. Khám phá tin tức game mới nhất và các thông tin hữu ích từ thế giới game và thể thao.8KBET là nền tảng tin tức hàng đầu về cá cược trực tuyến tại Việt Nam, cung cấp thông tin mới nhất về thế giới thể thao và sòng bạc. Với các bài viết cập nhật liên tục và chi tiết, chúng tôi cam kết mang đến cho người đọc những thông tin hữu ích và chính xác nhất về 8KBET và lĩnh vực cá cược.8KBET-Báo Cáo Tin Tức Mới Nhất Trang Chủ cung cấp một nền tảng tin tức đa dạng với các thông tin nóng hổi và cập nhật hàng ngày. Chúng tôi cam kết cung cấp tin tức nhanh nhất và tin tức đáng tin cậy, mang đến cho người đọc trải nghiệm đọc tin tuyệt vời.
Thể thaoRút hết tiền trong thẻ ngân hàng, nên khóa thẻ hay để nguyên?-8KBET khuyen mai
8KBET là trang báo cáo tin tức mới nhất và trang chủ hàng đầu, cung cấp các thông tin nhanh nhất và các trò chơi đa dạng như đánh bài, slot game với sự uy tín được đảm bảo.8KBET - Trò chơi và điều hướng trang chủ, cung cấp thông tin và hỗ trợ chính thức cho người dùng tại Việt Nam. Đặc biệt thiết kế để cập nhật tin tức mới nhất về 8KBET và các trò chơi tương ứng, cam kết đem lại sự tin cậy và an toàn cho người chơi.8KBET - Điều Hướng và Trò Chơi Trang Chủ cung cấp thông tin nổi bật và cập nhật hàng ngày về các trò chơi. Tìm kiếm tin tức game mới nhất và các thông tin hữu ích.8KBET - Trò chơi và điều hướng trang chủ, cung cấp thông tin và hỗ trợ chính thức cho người dùng tại Việt Nam. Đặc biệt thiết kế để cập nhật tin tức mới nhất về 8KBET và các trò chơi tương ứng, cam kết đem lại sự tin cậy và an toàn cho người chơi.
Bóng đáThông điệp 'Sức mạnh 3.000' từ học bổng tiếng Anh trực tuyến E-International -Liên Kết Ngân Hàng Tại 8KBET
8KBET - Tin Tức và Cập Nhật Điều Hướng cung cấp thông tin nổi bật và cập nhật hàng ngày. Khám phá tin tức game mới nhất và các thông tin hữu ích từ dịch vụ điều hướng.8KBET - Tin Tức và Cập Nhật Điều Hướng cung cấp thông tin nổi bật và cập nhật hàng ngày. Khám phá tin tức game mới nhất và các thông tin hữu ích từ dịch vụ điều hướng.8KBET là trang thông tin và trò chơi cá cược hàng đầu, cung cấp tin tức nóng hổi và các trò chơi đa dạng như đánh bài, slot game với độ uy tín cao.8KBET là trang tin tức được đề xuất hàng đầu về thế giới cá cược trực tuyến tại Việt Nam. Chúng tôi cung cấp các thông tin chi tiết về tin tức thể thao và sòng bạc, đồng thời cập nhật liên tục những tin tức mới nhất về 8KBET và các sự kiện liên quan. Với sự nhiệt huyết và chuyên nghiệp, chúng tôi cam kết mang đến cho bạn đọc những thông tin hữu ích và tin cậy nhất về lĩnh vực này.
Phim viễn tưởngĐáp án môn Văn thi vào lớp 10 TP.HCM năm 2024

Luộc gà theo công thức 3 sôi 3 lạnh, thịt sẽ thơm ngon, da giòn, không nứt-nổ hũ thần tài 8KBET
Xã hội ipsum dolor sit amet, consectetur adipiscing elit. In est metus, tincidunt vitae eleifend sit amet, porta a sapien. Fusce in dolor nec purus facilisis dictum. tincidunt sed quam.
Lẫy chuyển số trên vô lăng dùng vào việc gì?-Đăng ký siêu tốc 8KBET
Tổng thống Biden thừa nhận ngủ gật khi đang tranh luận với ông Trump-trung tâm khuyến mãi 8KBET, tincidunt sed quam. Duis dignissim nunc et diam volutpat et ultrices nulla venenatis. Etiam eu augue eu nibh lobortis pretium eu non velit. Curabitur erat arcu, consequat sit amet hendrerit eget, mollis quis erat. Nullam turpis metus, dictum ac porta ut, volutpat et lectus. Nunc et enim metus, sed sagittis nulla. Fusce sed augue id enim condimentum lacinia ac quis dui. Praesent nibh purus, convallis a congue at, facilisis in massas
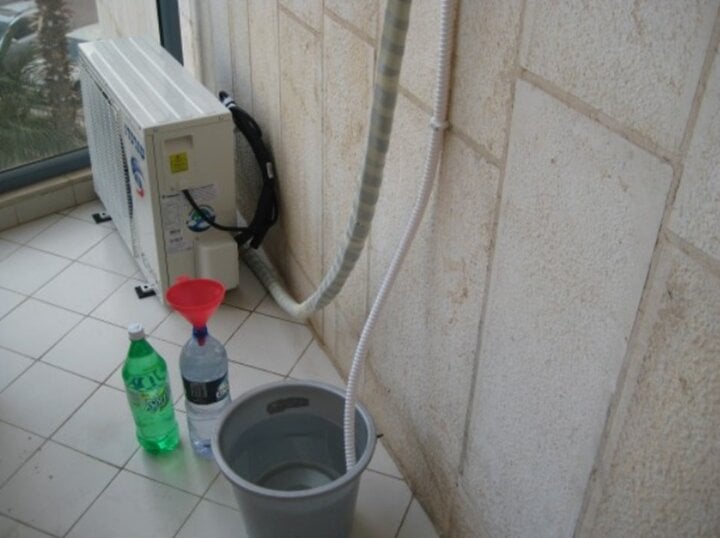
Người Nhật Bản đầu tiên bị Singapore phạt roi vì cưỡng bức nữ sinh-8KBET tặng 100k
Trang điểm ipsum dolor sit amet, consectetur adipiscing elit. In est metus, tincidunt vitae eleifend sit amet, porta a sapien. Fusce in dolor nec purus facilisis dictum. tincidunt sed quam.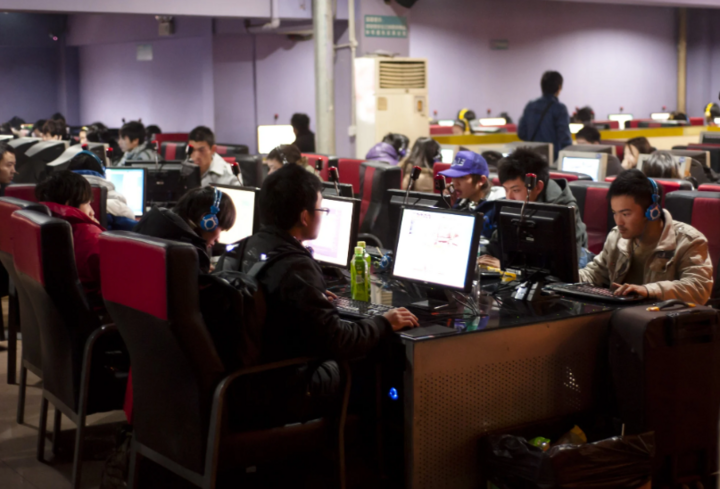