Hà Nội: Thay thế ngay cán bộ không có tâm thế phục vụ người dân, doanh nghiệp-Đăng ký khuyến mãi 8KBET
Công cụ trực tuyến được cung cấp miễn phí sẽ giúp người dùng tra cứu nhanh vị trí đặt chip NFC trên điện thoại để quét dữ liệu từ căn cước công dân.-Nổ hũ tỷ phú 8KBETHang pha lê ở Mexico nằm ở độ sâu 300 m dưới lòng đất, được mô tả là "đẹp như tranh vẽ" nhưng lại ẩn chứa nhiều yếu tố nguy hiểm chết người.-dang nhap 8KBETThác Dambri là một trong những điểm du lịch không thể bỏ lỡ khi bạn có dịp đến với Lâm Đồng.-nạp rút nhanh chóng 8KBET
Đua xeMột phụ nữ ở Hà Nội mất 1,2 tỷ đồng sau khi cài ứng dụng dịch vụ công giả-btl trong xổ số 8KBET là gì
Luật Thủ đô được thông qua có ý nghĩa lịch sử với nhiều quy định vượt trội, đột phá nhằm thúc đẩy xây dựng phát triển Thủ đô vươn tầm xứng đáng là đô thị đặc biệt.-8KBET bảo hiểm thua thể thaoHơn 100 cán bộ chiến sỹ công an kiểm tra đột xuất một quán bar ở Đắk Lắk, phát hiện hàng chục nam, nữ thanh niên có biểu hiện phê ma tuý.-cách vào 8KBET không bị virutVụ việc gây xôn xao dư luận mới diễn ra trước cổng một trường đại học ở Trung Quốc, cô nữ sinh cầm loa phóng thanh, livestream tố cáo kẻ xâm hại mình.-bắn cá nạp tiền 8KBET betTrong cuộc chiến chống tội phạm ma tuý, Thứ trưởng Bộ Công an đề nghị đấu tranh ngăn chặn nguồn cung, không để Việt Nam là địa bàn trung chuyển ma túy quốc tế.-8KBET nguyên nhân rút tiền bị chậm
Mẹo vặt làm đẹpBí mật tuyệt vời trên iPhone có thể bạn chưa biết-Phương pháp chơi rồng hổ 8KBET
Bài viết này sẽ cung cấp cho bạn những thông tin tư vấn chi tiết về xây dựng một ngôi nhà 3 tầng 4x18m2 đẹp, giúp bạn có được ngôi nhà mơ ước của mình.-Cập nhật trò chơi điện tử 8KBETBạn gái tôi thu nhập cao, có lúc tới 100 triệu đồng/tháng nhưng công việc không ổn định, lại làm ngày cày đêm, khiến tôi lo nếu cưới thì con cái sẽ khổ.-btl trong xổ số 8KBET là gìTrong phần bình luận trên sóng truyền hình, chuyên gia Phan Anh Tú cho rằng Quế Ngọc Hải chuyền bóng còn tốt hơn 2 trung vệ của đội tuyển Anh.-CSKH 8KBETNhững bãi đá ở danh thắng Bàn Than trải một màu xanh rì của những bãi rêu khiến du khách thích thú.-8KBETloto Casino Online
EconomyYêu nhầm nhân viên bất động sản, cô gái bị lừa mua nhà, nợ ngập mặt-Link Đăng ký bắn cá đổi thưởng 8KBET
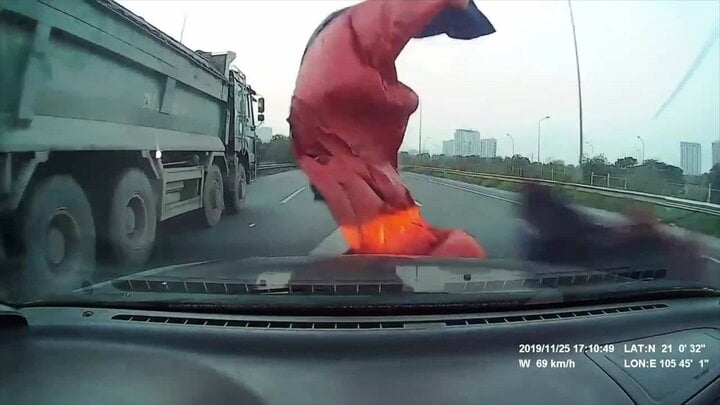
Mưa lũ ở Hà Giang làm 12 người chết và mất tích, thiệt hại trên 6 tỷ đồng-8KBET Bắt Cá Vũ Trụ
Môi trường ipsum dolor sit amet, consectetur adipiscing elit. In est metus, tincidunt vitae eleifend sit amet, porta a sapien. Fusce in dolor nec purus facilisis dictum. tincidunt sed quam.
Triệt phá đường dây cá độ bóng đá 150 tỷ đồng ở Nam Định-Đá Gà Trực Tiếp 8KBET
Đợt mưa lớn trút xuống miền Bắc kéo dài đến khi nào?-Bắn Cá CQ9 tại 8KBET, tincidunt sed quam. Duis dignissim nunc et diam volutpat et ultrices nulla venenatis. Etiam eu augue eu nibh lobortis pretium eu non velit. Curabitur erat arcu, consequat sit amet hendrerit eget, mollis quis erat. Nullam turpis metus, dictum ac porta ut, volutpat et lectus. Nunc et enim metus, sed sagittis nulla. Fusce sed augue id enim condimentum lacinia ac quis dui. Praesent nibh purus, convallis a congue at, facilisis in massas
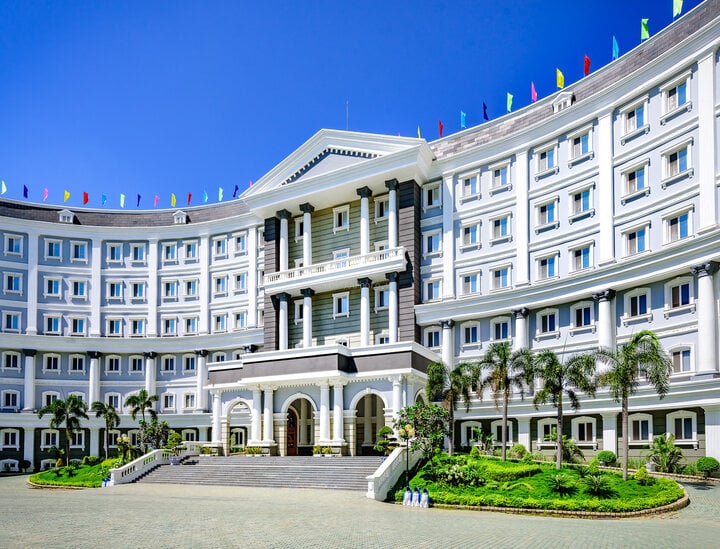