
IMAGE WITH DESCRIPTION
Điều gì xảy ra khi bạn ăn một quả chuối mỗi ngày?-cược thể thao 8KBET
Cười ipsum dolor sit amet, consectetur adipiscing elit. In est metus, tincidunt vitae eleifend sit amet, porta a sapien. Fusce in dolor nec purus facilisis dictum. tincidunt sed quam.
VIMEO VIDEO
6 cách đơn giản giúp bạn giảm cân 'ầm ầm' khi ngủ-Cách Hợp Tác Đại Lý 8KBET
Ngân hàng ipsum dolor sit amet, consectetur adipiscing elit. In est metus, tincidunt vitae eleifend sit amet, porta a sapien. Fusce in dolor nec purus facilisis dictum. tincidunt sed quam.
HTML5 MUSIC W/ FLASH FALLBACK
Nhận định EURO 2024: Sức mạnh đội hình, cơ hội vô địch của đội tuyển Pháp
Thủy sản ipsum dolor sit amet, consectetur adipiscing elit. In est metus, tincidunt vitae eleifend sit amet, porta a sapien. Fusce in dolor nec purus facilisis dictum. tincidunt sed quam.
YOUTUBE VIDEO
Gợi ý đáp án mã đề 118 môn Toán thi tốt nghiệp THPT 2024-kinh nghiệm rút tiền 8KBET
Y tế ipsum dolor sit amet, consectetur adipiscing elit. In est metus, tincidunt vitae eleifend sit amet, porta a sapien. Fusce in dolor nec purus facilisis dictum. tincidunt sed quam.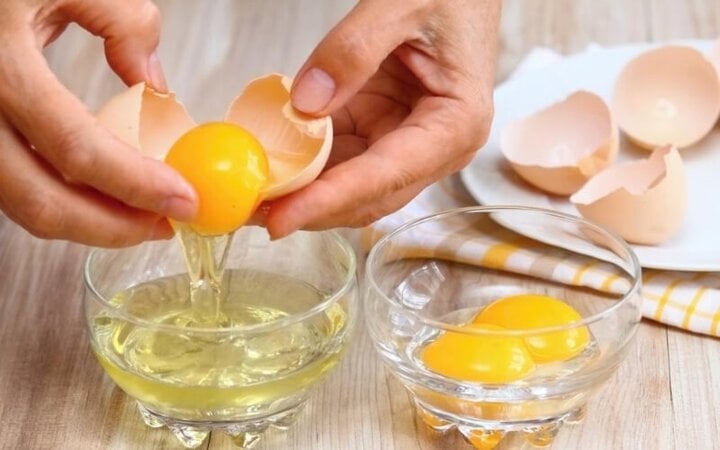
LOAD A FLASH FILE
Đề Văn lối mòn, thiếu đổi mới, sĩ tử dễ dàng đạt 7-8 điểm-Tin thể thao 8KBET
Sáng tạo ipsum dolor sit amet, consectetur adipiscing elit. In est metus, tincidunt vitae eleifend sit amet, porta a sapien. Fusce in dolor nec purus facilisis dictum. tincidunt sed quam.
TP.HCM: Giải mã sức hút nhà phố SOHO mặt tiền đường Đỗ Xuân Hợp-Trang chủ mới nhất 8KBET
Cụ bà 103 tuổi khỏe mạnh, minh mẫn nhờ 2 thói quen đơn giản này-8KBET ưu đãi cho thành viên mới, tincidunt sed quam. Duis dignissim nunc et diam volutpat et ultrices nulla venenatis. Etiam eu augue eu nibh lobortis pretium eu non velit. Curabitur erat arcu, consequat sit amet hendrerit eget, mollis quis erat. Nullam turpis metus, dictum ac porta ut, volutpat et lectus. Nunc et enim metus, sed sagittis nulla. Fusce sed augue id enim condimentum lacinia ac quis dui. Praesent nibh purus, convallis a congue at, facilisis in massas
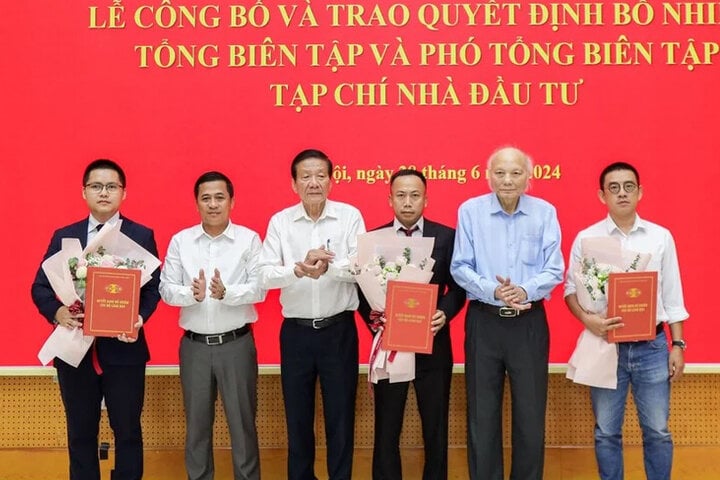
IMAGE WITH DESCRIPTION
Thường xuyên uống nước đậu đen rang có công dụng gì?-8KBET dowload
Thời trang trang sức ipsum dolor sit amet, consectetur adipiscing elit. In est metus, tincidunt vitae eleifend sit amet, porta a sapien. Fusce in dolor nec purus facilisis dictum. tincidunt sed quam.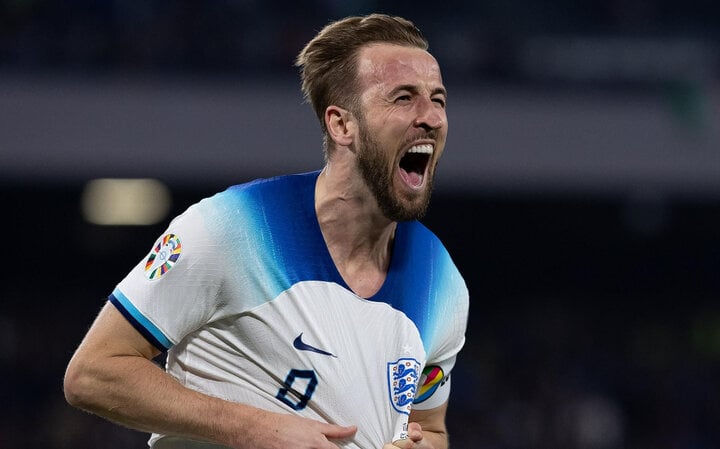
IMAGE WITH DESCRIPTION
TAND TP Hà Nội sắp xét xử vụ án xảy ra tại Tập đoàn FLC, trung tâm đăng kiểm-rút tiền 8KBET có an toàn không
Phim ảnh ipsum dolor sit amet, consectetur adipiscing elit. In est metus, tincidunt vitae eleifend sit amet, porta a sapien. Fusce in dolor nec purus facilisis dictum. tincidunt sed quam.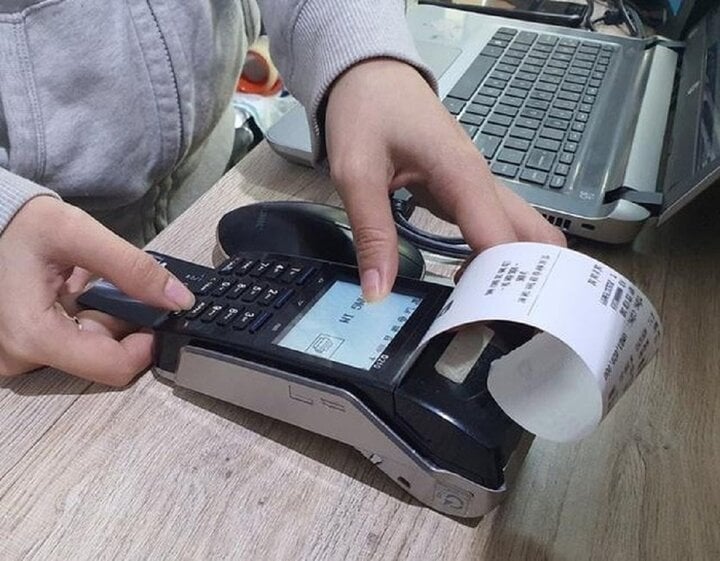
IMAGE WITH DESCRIPTION
Thứ trưởng Bộ Nội vụ Nguyễn Trọng Thừa nghỉ hưu từ 1/7-trang 8KBET lừa đảo
Trò chơi điện tử ipsum dolor sit amet, consectetur adipiscing elit. In est metus, tincidunt vitae eleifend sit amet, porta a sapien. Fusce in dolor nec purus facilisis dictum. tincidunt sed quam.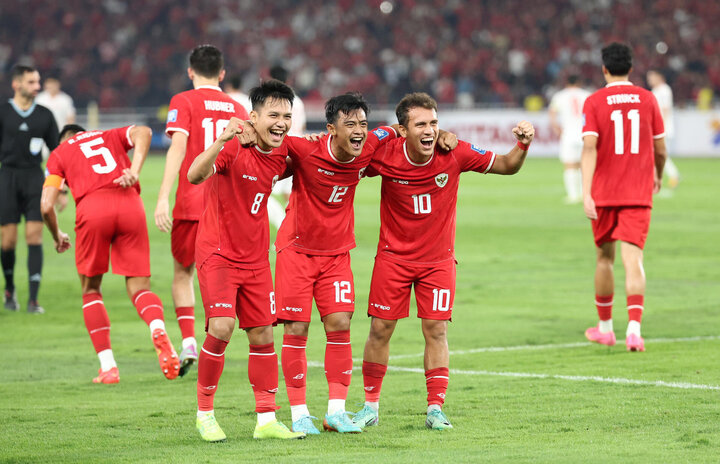
IMAGE WITH DESCRIPTION
Có nên cưới cô gái có khi kiếm 100 triệu đồng/tháng nhưng việc không ổn định?-btl trong xổ số 8KBET là gì
Pháp luật ipsum dolor sit amet, consectetur adipiscing elit. In est metus, tincidunt vitae eleifend sit amet, porta a sapien. Fusce in dolor nec purus facilisis dictum. tincidunt sed quam.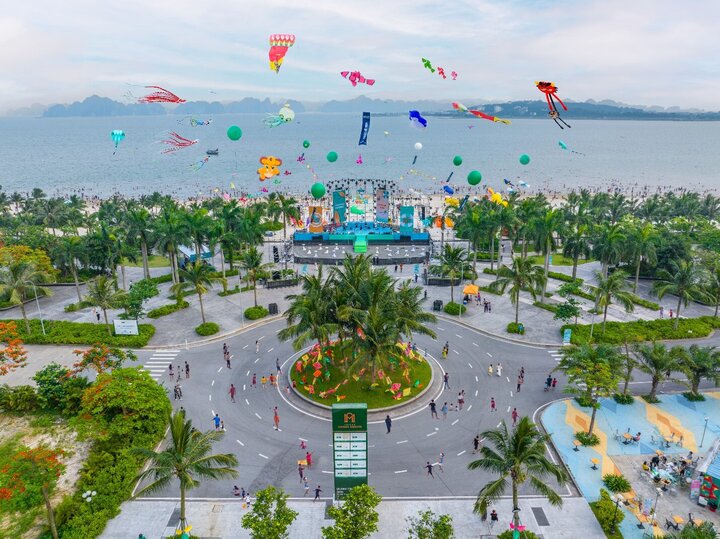
IMAGE WITH DESCRIPTION
Vụ đầu độc bằng xyanua ở Đồng Nai: Nạn nhân là cháu ruột nghi phạm-8KBET soi cầu hôm nay
Mẹo vặt làm đẹp ipsum dolor sit amet, consectetur adipiscing elit. In est metus, tincidunt vitae eleifend sit amet, porta a sapien. Fusce in dolor nec purus facilisis dictum. tincidunt sed quam.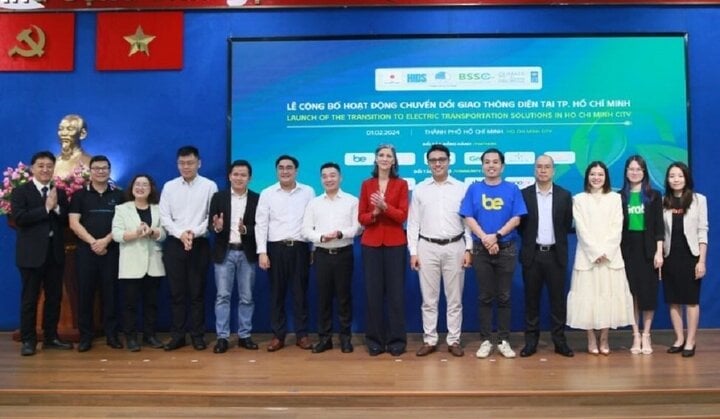
IMAGE WITH DESCRIPTION
Vàng 'chợ đen' giá cao ngất, dễ bán, khó mua-Điều hướng trang chủ 8KBET
Máy tính ipsum dolor sit amet, consectetur adipiscing elit. In est metus, tincidunt vitae eleifend sit amet, porta a sapien. Fusce in dolor nec purus facilisis dictum. tincidunt sed quam.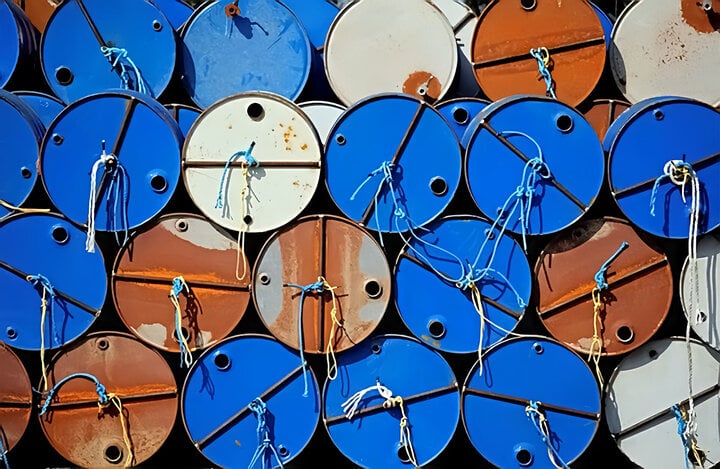
IMAGE WITH DESCRIPTION
Suni Hạ Linh lội ngược dòng vào chung kết 'Đạp gió 2024', Chi Pu nói gì?-Miễn trách nhiệm 8KBET
Nông nghiệp ipsum dolor sit amet, consectetur adipiscing elit. In est metus, tincidunt vitae eleifend sit amet, porta a sapien. Fusce in dolor nec purus facilisis dictum. tincidunt sed quam.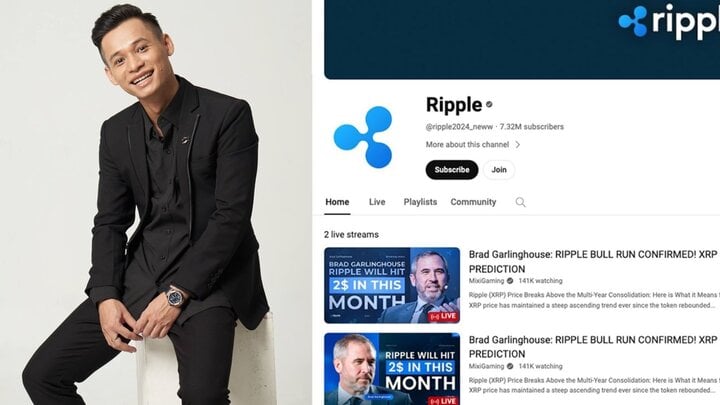
IMAGE WITH DESCRIPTION
Điểm tên nhà hàng Nhật Bản hút khách ở TP.HCM-Cập Nhật Thông Tin 8KBET
Internet ipsum dolor sit amet, consectetur adipiscing elit. In est metus, tincidunt vitae eleifend sit amet, porta a sapien. Fusce in dolor nec purus facilisis dictum. tincidunt sed quam.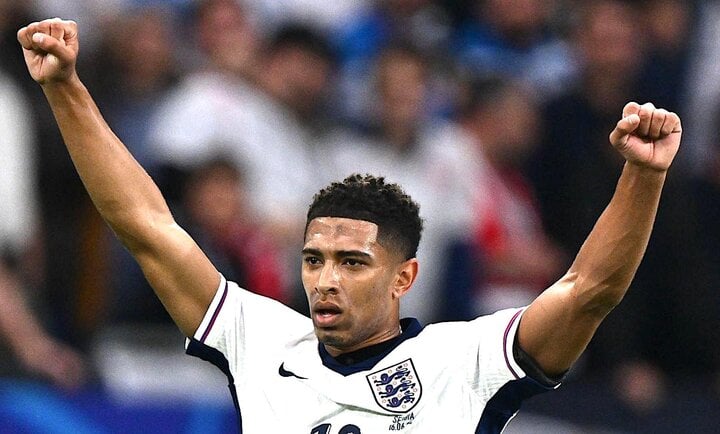
IMAGE WITH DESCRIPTION
Mãn nhãn với đội hình phản lực Yak-130 xé gió trên không-nhà cái 8KBET có uy tín không
Phim viễn tưởng ipsum dolor sit amet, consectetur adipiscing elit. In est metus, tincidunt vitae eleifend sit amet, porta a sapien. Fusce in dolor nec purus facilisis dictum. tincidunt sed quam.
IMAGE WITH DESCRIPTION
Tình tiết bất ngờ vụ tài xế taxi 'drift' gây náo loạn đường phố Hà Nội-8KBET ưu đãi cho thành viên mới
Món ngon mỗi ngày ipsum dolor sit amet, consectetur adipiscing elit. In est metus, tincidunt vitae eleifend sit amet, porta a sapien. Fusce in dolor nec purus facilisis dictum. tincidunt sed quam.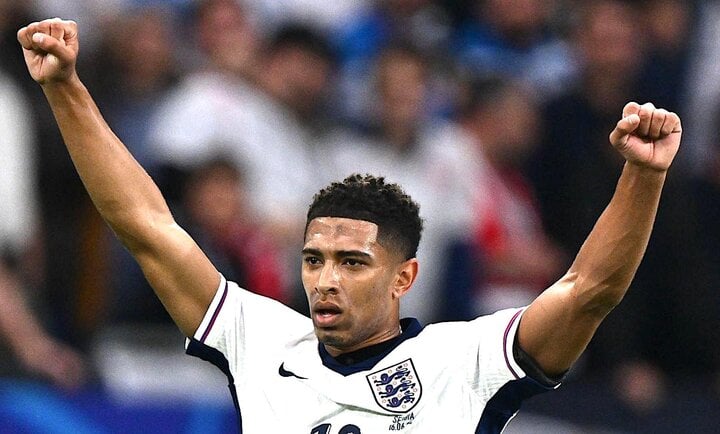
IMAGE WITH DESCRIPTION
Đáp án bài tổ hợp Khoa học Xã hội tốt nghiệp THPT 2024-8KBETB SA Gaming
Thể thao điện tử ipsum dolor sit amet, consectetur adipiscing elit. In est metus, tincidunt vitae eleifend sit amet, porta a sapien. Fusce in dolor nec purus facilisis dictum. tincidunt sed quam.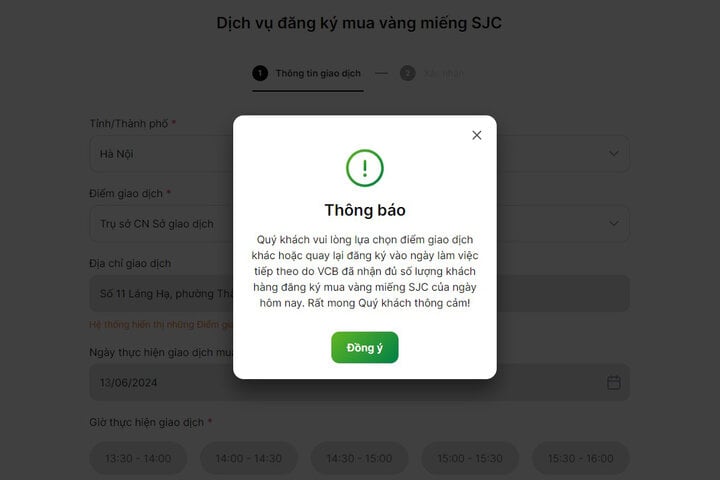
IMAGE WITH DESCRIPTION
Lên chức giám đốc, chồng tôi tăng tiền sinh hoạt phí, đưa cho vợ 8 triệu đồng-bắn cá đổi thưởng 8KBET
Cầu lông ipsum dolor sit amet, consectetur adipiscing elit. In est metus, tincidunt vitae eleifend sit amet, porta a sapien. Fusce in dolor nec purus facilisis dictum. tincidunt sed quam.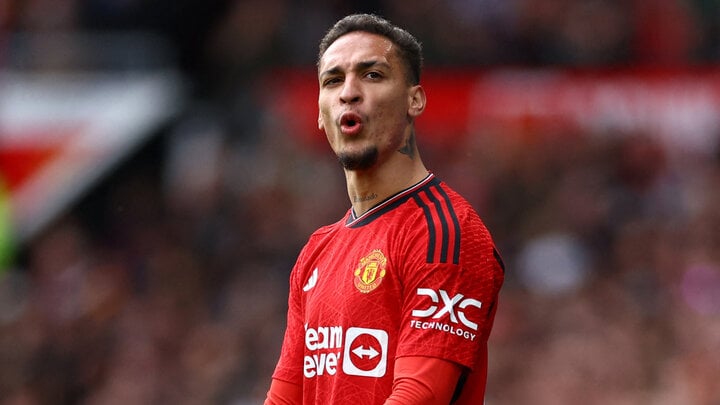
IMAGE WITH DESCRIPTION
Tổng thống Nga Putin thăm chính thức Việt Nam8KBET
Đổi mới ipsum dolor sit amet, consectetur adipiscing elit. In est metus, tincidunt vitae eleifend sit amet, porta a sapien. Fusce in dolor nec purus facilisis dictum. tincidunt sed quam.8KBET - Trò Chơi và Điều Hướng Mới Nhất cung cấp thông tin nổi bật và cập nhật hàng ngày về các trò chơi và thể thao. Tìm kiếm tin tức game mới nhất và các thông tin hữu ích.