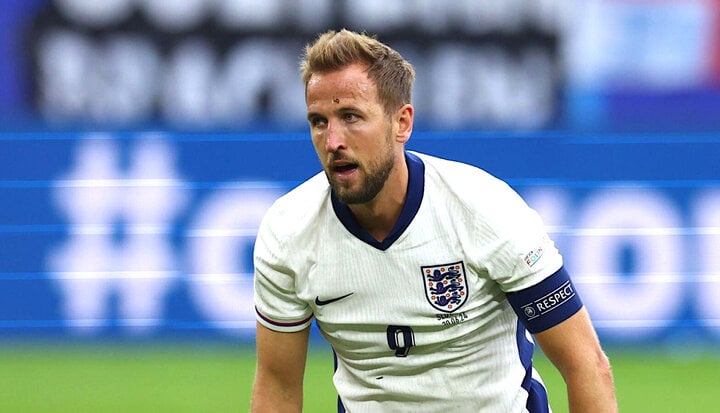
Tấn công mạng tống tiền điện tử ngày càng gia tăng, rất khó truy vết-Link tải game bắn cá 8KBET
Đàn ông đẹp ipsum dolor sit amet, consectetur adipiscing elit. In est metus, tincidunt vitae eleifend sit amet, porta a sapien. Fusce in dolor nec purus facilisis dictum. tincidunt sed quam.
Dự báo thời tiết 10 ngày tới: Sau mưa lớn, miền Bắc nắng nóng diện rộng-8KBET cập nhật 2024
Công nghệ thông tin ipsum dolor sit amet, consectetur adipiscing elit. In est metus, tincidunt vitae eleifend sit amet, porta a sapien. Fusce in dolor nec purus facilisis dictum. tincidunt sed quam.
Xác định CLB cuối cùng thăng hạng Premier League 2024/25-Tin Tức Sòng Bạc 8KBET
Xã hội ipsum dolor sit amet, consectetur adipiscing elit. In est metus, tincidunt vitae eleifend sit amet, porta a sapien. Fusce in dolor nec purus facilisis dictum. tincidunt sed quam.Cầu lông
8KBET - Tin tức trò chơi và điều hướng, cung cấp thông tin và hỗ trợ chính thức cho người dùng tại Việt Nam. Được thiết kế để cập nhật những tin tức mới nhất về 8KBET và các trò chơi, cam kết mang đến sự tin cậy và an toàn cho người chơi.8KBET là nền tảng tin tức hàng đầu về cá cược trực tuyến tại Việt Nam, cung cấp thông tin mới nhất về thế giới thể thao và sòng bạc. Với các bài viết cập nhật liên tục và chi tiết, chúng tôi cam kết mang đến cho người đọc những thông tin hữu ích và chính xác nhất về 8KBET và lĩnh vực cá cược.
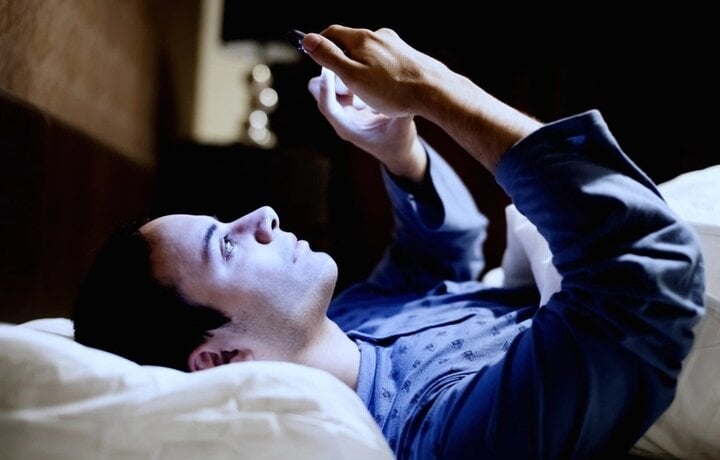
Mô hình kinh tế xanh trị giá tỷ USD được xây dựng tại Tây Ninh-cách lấy code 8KBET
Economy ipsum dolor sit amet, consectetur adipiscing elit. In est metus, tincidunt vitae eleifend sit amet, porta a sapien. Fusce in dolor nec purus facilisis dictum. tincidunt sed quam.
Trao tặng sổ BHXH, thẻ BHYT cho người dân có hoàn cảnh khó khăn-Thứ tự bài poker 8KBET
Thiết bị điện tử ipsum dolor sit amet, consectetur adipiscing elit. In est metus, tincidunt vitae eleifend sit amet, porta a sapien. Fusce in dolor nec purus facilisis dictum. tincidunt sed quam.
Chuyên gia Mỹ: Việt Nam là môi trường tuyệt vời cho bạn trẻ có đầu óc công nghệ-8KBET link mới
Âm nhạc ipsum dolor sit amet, consectetur adipiscing elit. In est metus, tincidunt vitae eleifend sit amet, porta a sapien. Fusce in dolor nec purus facilisis dictum. tincidunt sed quam.Khoa học
8KBET - Địa chỉ tin tức và dịch vụ chính hãng, cung cấp thông tin và hỗ trợ dành cho người dùng tại Việt Nam. Cập nhật tin tức mới nhất về 8KBET và các dịch vụ liên quan, đảm bảo uy tín và an toàn cho người chơi.8KBET là trang cập nhật trò chơi và điều hướng hàng đầu, cung cấp tin tức nhanh nhất và các trò chơi đa dạng như đánh bài, slot game với độ uy tín cao.

Dự báo thời tiết ngày mai 17/6: Bắc và Trung Bộ nắng rát, có nơi hơn 40 độ C-Trang Chủ Dịch Vụ Điều Hướng 8KBET Báo Cáo và Điều Hướng Đáng Tin Cậy
Cầu lông ipsum dolor sit amet, consectetur adipiscing elit. In est metus, tincidunt vitae eleifend sit amet, porta a sapien. Fusce in dolor nec purus facilisis dictum. tincidunt sed quam.
Cách kiểm tra vi phạm trên TikTok bằng điện thoại-Truy cập 8KBET
Trang điểm nam ipsum dolor sit amet, consectetur adipiscing elit. In est metus, tincidunt vitae eleifend sit amet, porta a sapien. Fusce in dolor nec purus facilisis dictum. tincidunt sed quam.
Hà Nội xem xét kỷ luật đảng viên liên quan gói thầu của AIC-Trò chơi 8KBET
Doanh nghiệp ipsum dolor sit amet, consectetur adipiscing elit. In est metus, tincidunt vitae eleifend sit amet, porta a sapien. Fusce in dolor nec purus facilisis dictum. tincidunt sed quam.Trang sức phụ kiện
8KBET - Tin tức điều hướng và báo cáo chính thức, cung cấp thông tin và hỗ trợ cho người dùng tại Việt Nam. Cập nhật tin tức mới nhất về 8KBET và các dịch vụ liên quan, cam kết đáp ứng các nhu cầu về tin cậy và an toàn cho người chơi.8KBET - Tin Tức và Cập Nhật Điều Hướng cung cấp thông tin nổi bật và cập nhật hàng ngày. Khám phá tin tức game mới nhất và các thông tin hữu ích từ dịch vụ điều hướng.
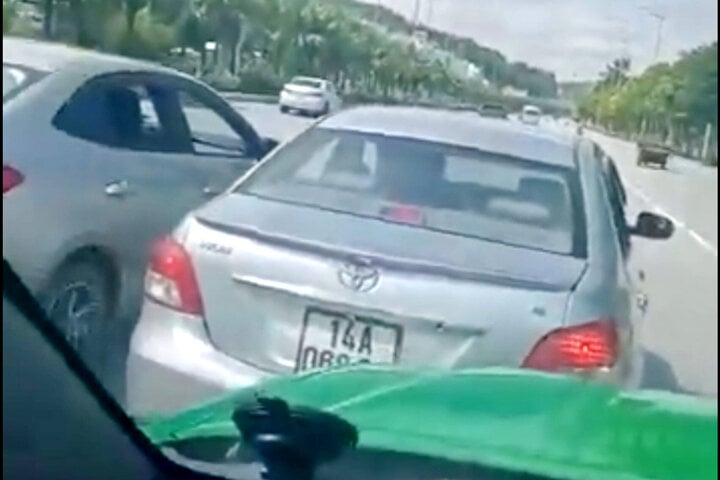
Phân loại rác tại nguồn ở Hải Phòng: Hàng loạt đơn vị chung tay-Web lô đề 8KBET
Ẩm thực quốc tế ipsum dolor sit amet, consectetur adipiscing elit. In est metus, tincidunt vitae eleifend sit amet, porta a sapien. Fusce in dolor nec purus facilisis dictum. tincidunt sed quam.
5 thói quen sống xanh từ những hành động đơn giản giúp bảo vệ môi trường-Bet 8KBET
Công nghệ ipsum dolor sit amet, consectetur adipiscing elit. In est metus, tincidunt vitae eleifend sit amet, porta a sapien. Fusce in dolor nec purus facilisis dictum. tincidunt sed quam.
Ca sĩ Giang Hồng Ngọc: Gia đình phá sản, chuyển 30 nhà trọ trong 17 năm-Đá gà thomo 8KBET
Chăm sóc da ipsum dolor sit amet, consectetur adipiscing elit. In est metus, tincidunt vitae eleifend sit amet, porta a sapien. Fusce in dolor nec purus facilisis dictum. tincidunt sed quam.Gia đình
8KBET - Trang Chủ Điều Hướng cung cấp thông tin nổi bật và cập nhật hàng ngày. Khám phá tin tức mới nhất và dịch vụ điều hướng chi tiết cho người dùng.8KBET - Tin Tức và Cập Nhật Điều Hướng cung cấp thông tin nổi bật và cập nhật hàng ngày. Khám phá tin tức game mới nhất và các thông tin hữu ích từ dịch vụ điều hướng.
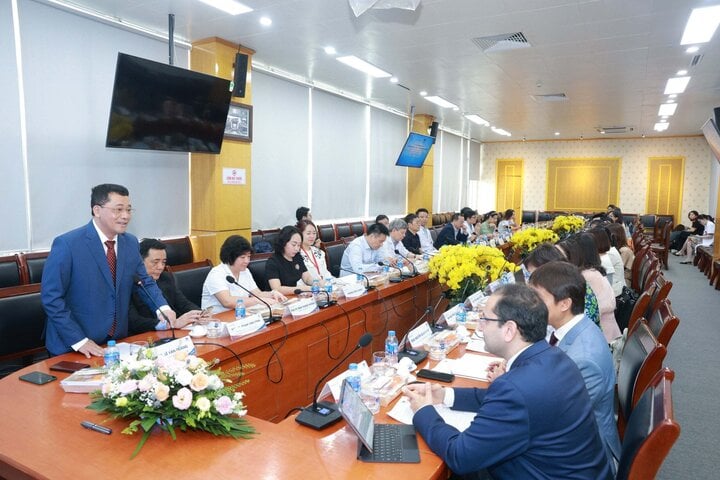
Đội tuyển Anh tập đá luân lưu trước vòng 1/8 EURO 2024-Trang Web Cá Độ Bóng Đá 8KBET
Golf ipsum dolor sit amet, consectetur adipiscing elit. In est metus, tincidunt vitae eleifend sit amet, porta a sapien. Fusce in dolor nec purus facilisis dictum. tincidunt sed quam.
Tuyển Anh thất bại 'muối mặt' trước thềm EURO 2024-8KBET asia
Nhảy múa ipsum dolor sit amet, consectetur adipiscing elit. In est metus, tincidunt vitae eleifend sit amet, porta a sapien. Fusce in dolor nec purus facilisis dictum. tincidunt sed quam.
Đề xuất thí điểm cho thuê vỉa hè quận Hoàn Kiếm cao nhất 240.000 đồng/m2/tháng-Đăng nhập game bài 8KBETb
Nhà đất ipsum dolor sit amet, consectetur adipiscing elit. In est metus, tincidunt vitae eleifend sit amet, porta a sapien. Fusce in dolor nec purus facilisis dictum. tincidunt sed quam.Ẩm thực quốc tế
8KBET - Tin tức trò chơi và điều hướng, cung cấp thông tin và hỗ trợ chính thức cho người dùng tại Việt Nam. Được thiết kế để cập nhật những tin tức mới nhất về 8KBET và các trò chơi, cam kết mang đến sự tin cậy và an toàn cho người chơi.8KBET-Tin Tức Mới Nhất và Điều Hướng cung cấp nền tảng tin tức đa dạng với các thông tin nóng hổi và cập nhật hàng ngày, bao gồm tin tức xã hội, tin tức thế giới, và tin tức đáng chú ý nhất. Với cam kết cập nhật nhanh nhất và tin tức đáng tin cậy, mang đến cho người đọc một trải nghiệm đọc tin tuyệt vời.
Hiphop
8KBET - Trò chơi mới nhất và tin tức, cung cấp thông tin và hỗ trợ chính thức cho người dùng tại Việt Nam. Sẵn sàng cập nhật những thông tin mới nhất về 8KBET và các trò chơi, cam kết đem lại sự tin cậy và an toàn cho người chơi.8KBET - Tin Tức và Cập Nhật Điều Hướng cung cấp thông tin nổi bật và cập nhật hàng ngày về các tin tức mới nhất và các thông tin thú vị từ thế giới game và thể thao.